Guessing Number Game with C#
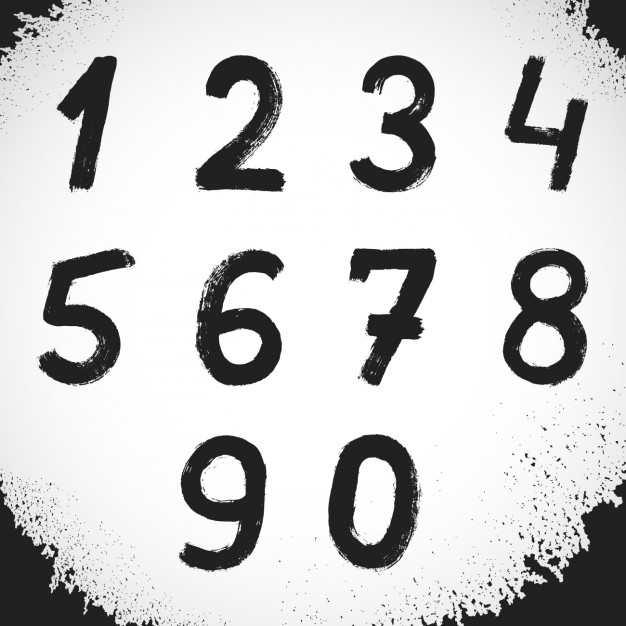
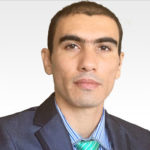
by Maihan Nijat
Guessing Number Game is not a famous or fun game but still widely used by instructors as an example or as an assignment.
In this tutorial, we will create a simple number guessing game with C#, using Visual Basic. The game is console base only – not UI.
Following are some rules for the game:
- Giving a user 7 chances to guess a randomly generated whole number between 1 and 100
- The program begins by randomly generating a number between 1 and 100
- The random number is winning number used to compare against users input
- The Game prompts user for input
Create a Solution
We start with creating Solution in Visual Basic. Open Visual Basic, create new Solution and name it: GuessingGame. Visual Basic automatically creates project structure including program.cs file which contains the following code by default:
program.cs
1 2 3 4 5 6 7 8 9 10 11 12 | using System; namespace GuessingGame { class MainClass { public static void Main(string[] args) { } } } |
Welcome Users and Create Variables
Let’s start with the editing program.cs file to add required code for making the game. All code goes inside the Main method.
Add the following line of code inside the Main method to welcome user:
1 | Console.WriteLine('Welcome to inputGuess Game!') |
Console.WriteLine(); is a method which prints on a screen. It accepts content as parameter.
We will create four properties/ variables as following:
1 2 3 4 | int randomNumber = new Random().Next(1, 101); int inputGuess; int countInputGuess = 0; Boolean userWin = false; |
The first variable generates a random number between 1 and 100.
The second variable store user’s input which is an integer.
The third variable keeps track of guesses. (How many times user guess?)
The last variable is a Boolean and by default the value is false. This is a flag for ending the game.
Creating playGame() Method
We create playGame() method which has while loop. The while loop runs until a user attempt is more than 7 or the game is won. The method has Console.ReadLine() which ask a user for input and then evaluate it with validateInput() method to make sure user entered the right option. It also calls evaluateAnswer() to determine a win, guess too low or guess too high.
playGame()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | void playGame() { while (countInputGuess != 7 && userWin != true) { Console.WriteLine('\nPlease enter your guess between 1 and 100!'); countInputGuess++; var userInput = Console.ReadLine(); if (validateInput(userInput)) { Console.WriteLine(evaluateAnswer()); } else { Console.WriteLine('Not a valid number! You also lost an attempt.'); } } } |
playGame() method is void, and we run it by calling it.
1 | playGame(); |
Validate User Input
It is important to check user’s input to make sure it’s an integer. validateInput() method returns Boolean after checking user’s input. It returns true if a user entered an integer, else false.
validateInput()
1 2 3 4 5 6 7 8 9 10 11 12 | Boolean validateInput(String userInput) { if (int.TryParse(userInput, out inputGuess)) { return true; } else { return false; } } |
Determine Win or Lost
Create evaluateAnswer() method which returns string base on win or loses situation. If a user wins: userWin value is changed to true and the game ends. The method also determines too low or too high guess and return strings to print on a screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | String evaluateAnswer() { if (inputGuess == randomNumber) { userWin = true; return $"YOU WIN. The number is { randomNumber } and your inputGuess is { inputGuess } :) \n"; } else if (countInputGuess == 7) { return $"\nYou Lost! You have tried { countInputGuess } times with no luck :( Random Number is { randomNumber } \n"; } else { if (inputGuess < randomNumber) { return $"Guess is too low! You have attempted { countInputGuess } times. \n"; } else { return $"Guess is too high! You have attempted { countInputGuess } times. \n"; } } } |
Create resetGame() Method and Loop Game
The last method is resetGame() which asks a user if want to play again or quit and reset variables with Y option.
resetGame()
1 2 3 4 5 6 7 8 9 10 11 | Boolean resetGame(){ Console.WriteLine("\nDo you want to play again? Y for yes"); userWin = false; countInputGuess = 0; if (Console.ReadKey().Key == ConsoleKey.Y) { return true; } else return false; } |
The last line is looping resetGame() method until a user chooses to quit the game.
1 2 3 4 | while(resetGame()) { playGame(); resetGame(); } |
Source Code
The complete source code is available on GitHub: GuessingNumber on GitHub
Recent Posts
Privacy Policy for Keyboard
April 22, 2024
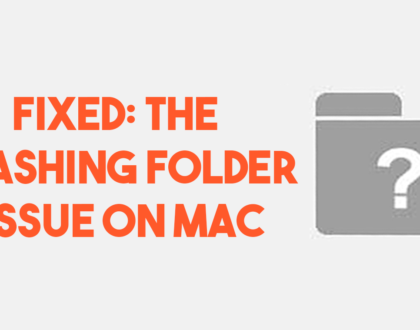
How to fix the MAC question mark folder issue?
August 3, 2021
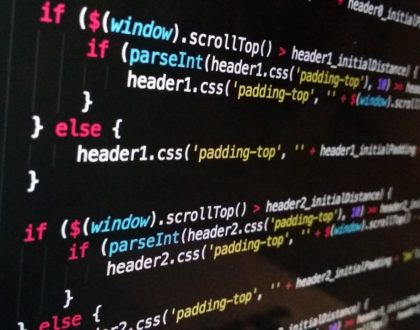
What is JavaScript Promise – Explained with examples?
March 23, 2021